Using the VALUE operator, you would be able to get the ITAB content assigned to the variable which is ITAB typed. Lets explore it in more details.
Preface
In ABAP 740, we have new VALUE operator to create the table entries. This VALUE operator works similarly to the NEW Operator to create the ITAB entries. Using the VALUE operator, the itab would be initialized and records would be inserted in the variable on the left side. Something like this:

Rule of thumb to use VALUE would be (same as NEW for ITAB):
Start a new row with opening bracket (,
Specify the name of the component and relevant value,
When done with that particular row finish it with closing bracket )
I have re-written all the example from the previous article on NEW operator for Itab here. I would omit all the explanation here as they are same as using the NEW. If you haven’t got a chance to read it through, please read it once.
There are few more variation to the VALUE operator and NEW operator, which we will try to explore in next article.
Difference between VALUE vs NEW
So you may ask what is the difference between VALUE vs NEW? Here are they:
- When you use NEW to create the Itab entries, you get the data reference back, where as when you use the VALUE operator, you get that data reference assigned to the variable on the left side.
- When using the NEW, the variable on the receiving side would be data reference TYPE REF TO DATA. Where as using the VALUE operator, the variable on the receiving side would be actual typed variable.
Example 1 – Standard table with Component as TABLE_LINE
Since I declared the variable using the TYPE for the itab, I can use the # to let system determine the type.
*---- * Example 1 *---- TYPES t_itab TYPE STANDARD TABLE OF i WITH DEFAULT KEY . * classical DATA itab_o TYPE t_itab. APPEND: 100 TO itab_o, 0 TO itab_o, 300 TO itab_o. * using VALUE - Variation 1 DATA(itab) = VALUE t_itab( ( 100 ) ( ) ( 300 ) ). * using VALUE - Variation 2 DATA itab_2 TYPE t_itab. itab_2 = VALUE #( ( 100 ) ( ) ( 300 ) ).
Debug compare:
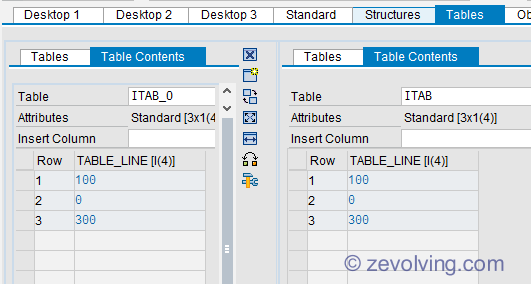
Example 2 – Sorted table with component as TABLE_LINE
Sorted table with TABLE_LINE as component
*---- * Example 2 *---- TYPES t_itab_sorted TYPE SORTED TABLE OF i WITH UNIQUE KEY table_line. * classical DATA itab_s_o TYPE t_itab_sorted. READ TABLE itab_s_o TRANSPORTING NO FIELDS WITH KEY table_line = 100. IF sy-subrc NE 0. INSERT 100 INTO itab_s_o INDEX sy-tabix. ENDIF. READ TABLE itab_s_o TRANSPORTING NO FIELDS WITH KEY table_line = 0. IF sy-subrc NE 0. INSERT 0 INTO itab_s_o INDEX sy-tabix. ENDIF. READ TABLE itab_s_o TRANSPORTING NO FIELDS WITH KEY table_line = 300. IF sy-subrc NE 0. INSERT 300 INTO itab_s_o INDEX sy-tabix. ENDIF. * using VALUE DATA(itab_sorted) = VALUE t_itab_sorted( ( 100 ) ( ) ( 300 ) ).
Debug compare output
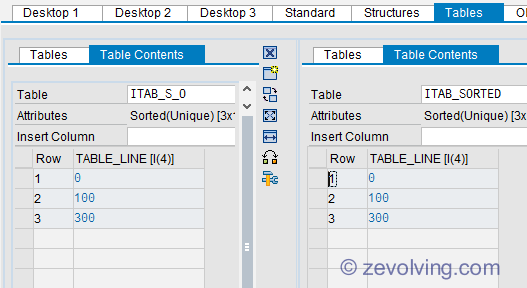
Example 3 – Sorted table with a specific component
Sorted table with Specific component
*---- * Example 3 *---- TYPES: BEGIN OF ty_sorted, num TYPE i, END OF ty_sorted, tt_sorted TYPE SORTED TABLE OF ty_sorted WITH UNIQUE KEY num. * Using VALUE DATA(itab_sorted_c) = VALUE tt_sorted( ( num = 100 ) ( ) ( num = 300 ) ).
Example 4 – Table with more components
Standard table with more components
* Example 4 TYPES: BEGIN OF ty_data, kunnr TYPE kunnr, name1 TYPE name1, ort01 TYPE ort01, land1 TYPE land1, END OF ty_data. TYPES: tt_data TYPE STANDARD TABLE OF ty_data WITH DEFAULT KEY. * classical DATA: itab_multi_c TYPE tt_data. FIELD-SYMBOLS: <fs> LIKE LINE OF itab_multi_c. APPEND INITIAL LINE TO itab_multi_c ASSIGNING <fs>. <fs>-kunnr = '123'. <fs>-name1 = 'ABCD'. <fs>-ort01 = 'LV'. <fs>-land1 = 'NV'. APPEND INITIAL LINE TO itab_multi_c ASSIGNING <fs>. <fs>-kunnr = '456'. <fs>-name1 = 'XYZ'. <fs>-ort01 = 'LA'. <fs>-land1 = 'CA'. * Using VALUE DATA(itab_multi_comp) = VALUE tt_data( ( kunnr = '123' name1 = 'ABCD' ort01 = 'LV' land1 = 'NV' ) ( kunnr = '456' name1 = 'XYZ' ort01 = 'LA' land1 = 'CA' ) ).
Debug compare output
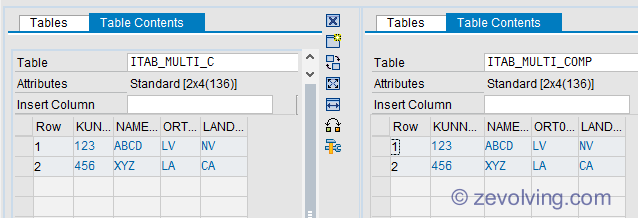
Example 5 – Table with Deep structure
Standard table with deep structure
* Example 5 TYPES: BEGIN OF ty_alv_data, kunnr TYPE kunnr, name1 TYPE name1, ort01 TYPE ort01, land1 TYPE land1, t_color TYPE lvc_t_scol, END OF ty_alv_data. TYPES: tt_alv_data TYPE STANDARD TABLE OF ty_alv_data WITH DEFAULT KEY. * classical DATA: itab_alv_c TYPE tt_alv_data. FIELD-SYMBOLS: <fs_a> LIKE LINE OF itab_alv_c, <fs_col> LIKE LINE OF <fs_a>-t_color. APPEND INITIAL LINE TO itab_alv_c ASSIGNING <fs_a>. <fs_a>-kunnr = '123'. <fs_a>-name1 = 'ABCD'. <fs_a>-ort01 = 'LV'. <fs_a>-land1 = 'NV'. APPEND INITIAL LINE TO <fs_a>-t_color ASSIGNING <fs_col>. <fs_col>-fname = 'KUNNR'. <fs_col>-color-col = col_negative. <fs_col>-color-int = 0. <fs_col>-color-inv = 0. APPEND INITIAL LINE TO <fs_a>-t_color ASSIGNING <fs_col>. <fs_col>-fname = 'ORT01'. <fs_col>-color-col = col_total. <fs_col>-color-int = 1. <fs_col>-color-inv = 1. APPEND INITIAL LINE TO itab_alv_c ASSIGNING <fs_a>. <fs_a>-kunnr = '456'. <fs_a>-name1 = 'XYZ'. <fs_a>-ort01 = 'LA'. <fs_a>-land1 = 'CA'. * Using VALUE DATA(itab_alv) = VALUE tt_alv_data( "First Row ( kunnr = '123' name1 = 'ABCD' ort01 = 'LV' land1 = 'NV' " color table t_color = VALUE #( " Color table - First Row ( fname = 'KUNNR' color-col = col_negative color-int = 0 color-inv = 0 ) " Color Table - 2nd Row ( fname = 'ORT01' color-col = col_total color-int = 1 color-inv = 1 ) ) ) "Second row ( kunnr = '456' name1 = 'XYZ' ort01 = 'LA' land1 = 'CA' ) ).
Debug compare output
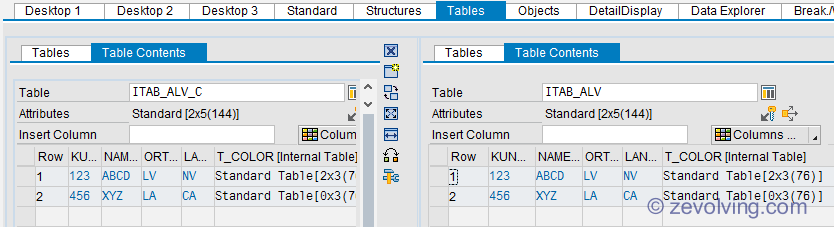
What do you think?
I think I would use VALUE operator more than NEW operator, as I don’t need to use the helper variables – field symbols – to access them later. What do you think?
Table of Content – ABAP 740 Concepts
- ABAP 740 – NEW Operator to instantiate the objects
- ABAP 740 – NEW Operator to create ITAB entries
- ABAP 740 – VALUE Operator to create ITAB entries
- ABAP 740 – Table Expressions to Read & Modify ITAB line
- ABAP 740 – LINE_EXISTS to check record in ITAB
- ABAP 740 – Meshes – A new complex type of Structures
- ABAP 740 – Mesh Path – Forward and Inverse Association
- ABAP 740 – FOR Iteration Expression
- ABAP 740 SWITCH – Conditional Operator
- ABAP 740 – LOOP AT with GROUP BY
- ABAP 740 – Is CONSTANT not a Static Attribute anymore?
- SALV IDA (Integrated Data Access) – Introduction
- SALV IDA – Selection Conditions
- SALV IDA – New Calculated Fields
- SALV IDA – Column Settings
- SALV IDA – Add and Handle Hotspot (Hyperlink)
Hallo Naimesh,
it is really very nice, but what do you think,. which is better this new version.. or classic, I mean ur opinion.
thank you very much.
I’m still searching for valid use cases for the VALUES operator.
Currently I only can imaging using it for
– drop down lists (but should be otherwise solved IMO)
– Mock Server / Unit Tests
What do you think?
Hello Uwe,
I guess I’m also in the same boat – searching for the use case for both NEW and VALUE for ITAB.
It would be useful in
– ABAP Unit test (as you mentioned)
– Fill up the ranges for specific internal document type like O, M, J etc; Partner function
Thanks.
Hi Naimesh,
Thanks for sharing this. The value operator would be useful for writing key value pairs for picklists (Dropdown lists) in WebUI. It would reduce the number APPEND statements for the table.
Regards,
Mahesh