SWITCH usage with returning the object reference. This would be great to reduce lot of clutter from the design pattern implementation i.e. Factory design pattern.
Preface
In the last article ABAP 740 SWITCH – Conditional Operator, I mentioned – the returned value is the object reference, the up-casting should be possible. This means that the Switch is capable of handling the object reference. Let see how.
Factory Design pattern using Switch
A while back, I demonstrated the implementation of the Factory Design patterns in ABAP objects. This was using the simple IF, ELSE. To recap, the Factory method design pattern hides all the complexity of object creation from the consumer of the object. Its good idea to read the article Factory Design patterns in ABAP objects before moving further.
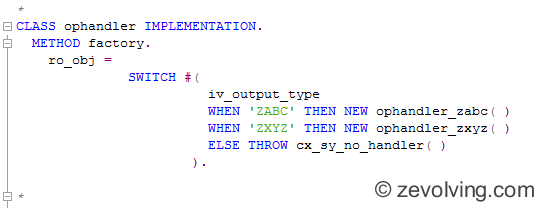
Now, with ABAP 740 – we can use the SWITCH instead of the IF .. ELSE .. ENDIF
Factory Design pattern Using SWITCH
*===== CLASS ophandler DEFINITION ABSTRACT. PUBLIC SECTION. CLASS-METHODS: factory IMPORTING iv_output_type TYPE kschl RETURNING VALUE(ro_obj) TYPE REF TO ophandler. METHODS: process_output ABSTRACT. ENDCLASS. "ophandler DEFINITION *===== CLASS ophandler_zabc DEFINITION INHERITING FROM ophandler. PUBLIC SECTION. METHODS: process_output REDEFINITION. ENDCLASS. "ophandler_zabc DEFINITION * CLASS ophandler_zabc IMPLEMENTATION. METHOD process_output. WRITE: / 'Processing ZABC'. ENDMETHOD. "process_output ENDCLASS. "ophandler_zabc IMPLEMENTATION *===== CLASS ophandler_zxyz DEFINITION INHERITING FROM ophandler. PUBLIC SECTION. METHODS: process_output REDEFINITION. ENDCLASS. * CLASS ophandler_zxyz IMPLEMENTATION. METHOD process_output. WRITE: / 'Processing ZXYZ'. ENDMETHOD. "process_output ENDCLASS. * CLASS ophandler IMPLEMENTATION. METHOD factory. ro_obj = SWITCH #( iv_output_type WHEN 'ZABC' THEN NEW ophandler_zabc( ) WHEN 'ZXYZ' THEN NEW ophandler_zxyz( ) ELSE THROW cx_sy_no_handler( ) ). * * CASE iv_output_type. * WHEN 'ZABC'. * CREATE OBJECT ro_obj TYPE ophandler_zabc. * WHEN 'ZXYZ'. * "create another object * WHEN OTHERS. * " raise exception * ENDCASE. ENDMETHOD. "factory ENDCLASS. "ophandler IMPLEMENTATION *===== CLASS lcl_main_app DEFINITION. PUBLIC SECTION. CLASS-METHODS: run. ENDCLASS. "lcl_main_app DEFINITION * CLASS lcl_main_app IMPLEMENTATION. METHOD run. DATA: lo_output TYPE REF TO ophandler. lo_output = ophandler=>factory( 'ZABC' ). lo_output->process_output( ). ENDMETHOD. "run ENDCLASS. "lcl_main_app IMPLEMENTATION START-OF-SELECTION. lcl_main_app=>run( ).
Table of Content – Design Patterns
Explore all articles in ABAP Design Patterns
- ABAP Object Design Patterns – Singleton
- ABAP Objects Design Patterns – Model View Controller (MVC) Part 1
- ABAP Objects Design Patterns – Model View Controller (MVC) Part 2
- ABAP Objects Design Patterns – Model View Controller (MVC) Part 3
- ABAP Objects Design Patterns – Decorator
- ABAP Objects Design Patterns – Factory Method
- ABAP Objects Design Patterns – Observer
- Case Study: Observer Design Pattern Usage
- ABAP Objects Design Patterns – Abstract Factory
- OO Design Pattern Decorator – Why do we need to use helper variable?
- ABAP Objects Design Patterns – Facade
- ABAP Objects Design Patterns – Adapter
- ABAP Objects Design Patterns – Composite
- ABAP Objects Design Patterns – Iterator
- Iterator Design Pattern to access Linked List in ABAP Objects
- ABAP Objects Design Patterns – Proxy
- ABAP Objects Design Patterns – Prototype
- ABAP Objects Design Patterns – Singleton Factory
- ABAP Object Oriented Approach for Reports – Initial Design
- ABAP Object Oriented Approach for Reports – Redesign
- ABAP Objects Design Patterns – Builder
- ABAP Objects Design Patterns Singleton Usage
- ABAP MVC – Model View Controller Discussion
- Object Oriented Approach for Reports with multiple Datasource
- OO ABAP – Selection Object with Direct Access
- OO ABAP – Selection Criteria Object using RS_REFRESH_FROM_SELECT OPTIONS
- ABAP Factory Method Using SWITCH
Nice !
I will try to apply it as soon as possible and, for sure, it’s very appreciated you keep us up to date =)
Simply Excellent Article.
A very helpful post! Thanks for sharing!
For more information on SAP ABAP services you can visit http://www.apprisia.com/sap-r3/sap-abap-development-services/