As name suggests, this new predictive function checks if the Line is available in the internal table or not.
Introduction
LINE_EXISTS can be used instead of the Table Expressions.
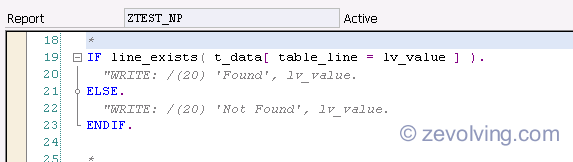
Here are the few things to consider:
- LINE_EXISTS is same as READ TABLE … TRANSPORTING NO FIELDS .. followed by SY-SUBRC CHECK.
- The call doesn’t return any value. It only checks if the line is there in the table with specified key or not
- This Function doesn’t set the system field SY-TABIX. So, this can’t be used if you want to use the Parallel Cursor. So, I think you would need use still use the old READ TABLE statement.
- From SAP Help, This function should be used carefully – you should not use this function to first check if the line is there in the table and than use the table expression to read the table. Instead of that, only the table expression assigning to Field Symbol followed by catching the exception as you did in the article ABAP 740 – Table Expressions to Read & Modify ITAB line, example 5
When to use LINE_EXISTS
As mentioned earlier, it can only replace few instanced of the READ TABLE… TRANSPORTING NO FIELDS which are not using the result value. If you want the result value, use the Table expression instead. If you want to use the SY-TABIX, you would need to use the old READ TABLE statement. I got excite to replace my parallel cursor algorithms but I think I would need to halt on that 🙁
Lets see few example.
Example – Simple Usage with SY fields behavior
Check if the line exist with the value which exists in the table, followed by the entry which doesn’t exist with equivalent old code.
DATA: t_data TYPE STANDARD TABLE OF i. DATA(lv_value) = 9. DO 10 TIMES. APPEND sy-index TO t_data. ENDDO. * WRITE: / '>>>> New', lv_value. IF line_exists( t_data[ table_line = lv_value ] ). WRITE: /(20) 'Found', lv_value. ELSE. WRITE: /(20) 'Not Found', lv_value. ENDIF. WRITE: /(20) 'SUBRC', sy-subrc, /(20) 'TABIX', sy-tabix, /(20) 'INDEX', sy-index. * lv_value = 15. WRITE: / '>>> New', lv_value. IF line_exists( t_data[ table_line = lv_value ] ). WRITE: /(20) 'Found', lv_value. ELSE. WRITE: /(20) 'Not Found', lv_value. ENDIF. WRITE: /(20) 'SUBRC', sy-subrc, /(20) 'TABIX', sy-tabix, /(20) 'INDEX', sy-index. * old WRITE: /'<<<< OLD', lv_value. READ TABLE t_data TRANSPORTING NO FIELDS WITH KEY table_line = lv_value. IF sy-subrc EQ 0. WRITE: /(20) 'Found', lv_value. ELSE. WRITE: /(20) 'Not Found', lv_value. ENDIF. WRITE: /(20) 'SUBRC', sy-subrc, /(20) 'TABIX', sy-tabix, /(20) 'INDEX', sy-index. [/abap_code] As you can notice from the output; SY-TABIX, SY-INDEX, SY-SUBRC doesn't reset from the LINE_EXISTS call. <div class="post-image"><img loading="lazy" src="http://zevolving.com/wp-content/uploads/2015/03/ABAP_740_Line_Exists_Example_1.png" alt="ABAP_740_Line_Exists_Example_1" width="410" height="382" class="alignnone size-full wp-image-2846" srcset="http://zevolving.com/wp-content/uploads/2015/03/ABAP_740_Line_Exists_Example_1.png 410w, http://zevolving.com/wp-content/uploads/2015/03/ABAP_740_Line_Exists_Example_1-300x279.png 300w" sizes="(max-width: 410px) 100vw, 410px" /></div> <h3>Example - Simple Negative Usage with SY fields behavior</h3> Negative check using NOT and NE .. [abap_code slider="X" title=""] * lv_value = 15. WRITE: / '>>> New', lv_value. IF NOT line_exists( t_data[ table_line = lv_value ] ). WRITE: /(20) 'Not Found', lv_value. ELSE. WRITE: /(20) 'Found', lv_value. ENDIF. * old WRITE: /'<<<< OLD', lv_value. READ TABLE t_data TRANSPORTING NO FIELDS WITH KEY table_line = lv_value. IF sy-subrc NE 0. WRITE: /(20) 'Not Found', lv_value. ELSE. WRITE: /(20) 'Found', lv_value. ENDIF. [/abap_code] <div class="post-image"><img loading="lazy" src="http://zevolving.com/wp-content/uploads/2015/03/ABAP_740_Line_Exists_Example_2.png" alt="ABAP_740_Line_Exists_Example_2" width="413" height="203" class="alignnone size-full wp-image-2847" srcset="http://zevolving.com/wp-content/uploads/2015/03/ABAP_740_Line_Exists_Example_2.png 413w, http://zevolving.com/wp-content/uploads/2015/03/ABAP_740_Line_Exists_Example_2-300x147.png 300w" sizes="(max-width: 413px) 100vw, 413px" /></div> <h3>Example - Compare with Table Expression and LINE_EXISTS and READ</h3> [abap_code slider="X" title=""] * New function lv_value = 15. WRITE: / '>>> New', lv_value. IF NOT line_exists( t_data[ table_line = lv_value ] ). WRITE: /(20) 'Not Found', lv_value. ELSE. WRITE: /(20) 'Found', lv_value. ENDIF. * New Way TRY . lv_value = 7. WRITE: / '>>> New with Table Expression', lv_value. DATA(lv_num) = t_data[ table_line = lv_value ]. WRITE: /(20) 'SUBRC', sy-subrc, /(20) 'TABIX', sy-tabix, /(20) 'INDEX', sy-index. CATCH cx_sy_itab_line_not_found. WRITE: / 'Not found'. ENDTRY. * old WRITE: /'<<<< OLD', lv_value. READ TABLE t_data TRANSPORTING NO FIELDS WITH KEY table_line = lv_value. IF sy-subrc NE 0. WRITE: /(20) 'Not Found', lv_value. ELSE. WRITE: /(20) 'Found', lv_value. ENDIF. [/abap_code] Even table expression don't reset the SY-TABIX field ... <div class="post-image"><img loading="lazy" src="http://zevolving.com/wp-content/uploads/2015/03/ABAP_740_Line_Exists_Example_3.png" alt="ABAP_740_Line_Exists_Example_3" width="403" height="285" class="alignnone size-full wp-image-2848" srcset="http://zevolving.com/wp-content/uploads/2015/03/ABAP_740_Line_Exists_Example_3.png 403w, http://zevolving.com/wp-content/uploads/2015/03/ABAP_740_Line_Exists_Example_3-300x212.png 300w" sizes="(max-width: 403px) 100vw, 403px" /></div> <h3>Example - Multiple Component check</h3> LINE_EXISTS works with simple multiple component check as well. [abap_code slider="X" title=""] * Multiple Components TYPES: BEGIN OF ty_data, kunnr TYPE kunnr, name1 TYPE name1, ort01 TYPE ort01, land1 TYPE land1, END OF ty_data. TYPES: tt_data TYPE STANDARD TABLE OF ty_data WITH DEFAULT KEY. * DATA(itab_multi_comp) = VALUE tt_data( ( kunnr = '123' name1 = 'ABCD' ort01 = 'LV' land1 = 'NV' ) ( kunnr = '456' name1 = 'XYZ' ort01 = 'LA' land1 = 'CA' ) ). WRITE: / 'NEW'. IF line_exists( itab_multi_comp[ kunnr = '456' ] ). WRITE: / ' Customer found'. ELSE. WRITE: / ' Customer Not found'. ENDIF. * old WRITE: / 'OLD'. READ TABLE itab_multi_comp TRANSPORTING NO FIELDS WITH KEY kunnr = '456'. IF sy-subrc EQ 0. WRITE: / ' Customer found'. ELSE. WRITE: / ' Customer Not found'. ENDIF.
Output
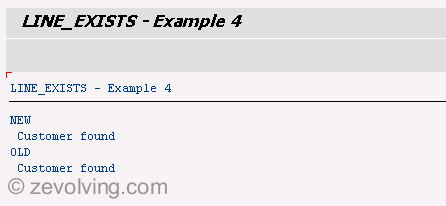
Example – Chaining of Table Expression within LINE_EXISTS now allowed
* Itab Deep TYPES: BEGIN OF ty_alv_data, kunnr TYPE kunnr, name1 TYPE name1, ort01 TYPE ort01, land1 TYPE land1, t_color TYPE lvc_t_scol, END OF ty_alv_data. TYPES: tt_alv_data TYPE STANDARD TABLE OF ty_alv_data WITH DEFAULT KEY. DATA(itab_alv) = VALUE tt_alv_data( "First Row ( kunnr = '123' name1 = 'ABCD' ort01 = 'LV' land1 = 'NV' " color table t_color = VALUE #( " Color table - First Row ( fname = 'KUNNR' color-col = col_negative color-int = 0 color-inv = 0 ) " Color Table - 2nd Row ( fname = 'ORT01' color-col = col_total color-int = 1 color-inv = 1 ) ) ) "Second row ( kunnr = '456' name1 = 'XYZ' ort01 = 'LA' land1 = 'CA' ) ). if line_exists( itab_alv[ kunnr = '123' ]-t_color[ fname = 'ORT01' ]-color-col = col_background ). endif.
This produces the syntax error that it doesn’t like it ..
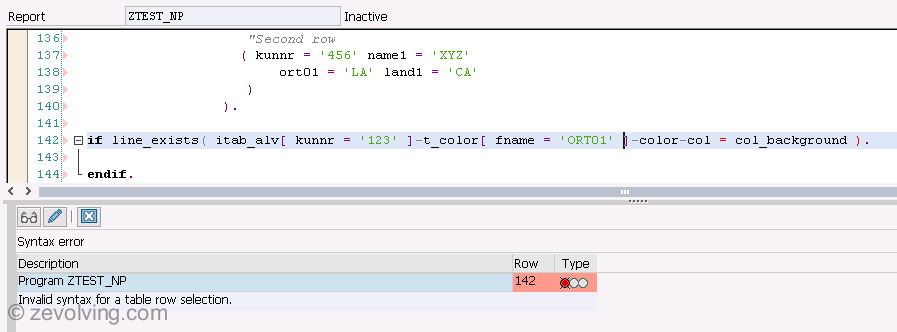
What do you think?
I think I would more use the READ TABLE as generally need to get the access to SY-TABIX for parallel cursor.
Table of Content – ABAP 740 Concepts
- ABAP 740 – NEW Operator to instantiate the objects
- ABAP 740 – NEW Operator to create ITAB entries
- ABAP 740 – VALUE Operator to create ITAB entries
- ABAP 740 – Table Expressions to Read & Modify ITAB line
- ABAP 740 – LINE_EXISTS to check record in ITAB
- ABAP 740 – Meshes – A new complex type of Structures
- ABAP 740 – Mesh Path – Forward and Inverse Association
- ABAP 740 – FOR Iteration Expression
- ABAP 740 SWITCH – Conditional Operator
- ABAP 740 – LOOP AT with GROUP BY
- ABAP 740 – Is CONSTANT not a Static Attribute anymore?
- SALV IDA (Integrated Data Access) – Introduction
- SALV IDA – Selection Conditions
- SALV IDA – New Calculated Fields
- SALV IDA – Column Settings
- SALV IDA – Add and Handle Hotspot (Hyperlink)
Hi, is it possible to use this together with other key than whole TABLE LINE? Otherwise I see no sense to remember it, because TRANSPORTING NO FIELDS, as you mentioned, has much bigger area of use, and yes, no SY-TABIX is also awkward.
Hello Martin,
Yes, it does work with the Multiple components. I have added the example in the article for that now. I was more focused on the SY fields so didn’t focus on all other cases.
Also added chaining of the table expression example which is not yet allowed using LINE_EXISTS. May be in next release 🙂
Thanks,
Naimesh Patel
Hi Naimesh,
I think the reason why line_exists does not work is stated in the documentation:
The table expression is only used to check the existence of the specified row. No temporary result is created.
To modify the deep component, the chaining table expressions can be used together with the exception handling (catching the exception is the same as line_exists = false).
Check the snippet below:
BR
Michal
Oh, I can see that you already described the same here, sorry:
http://zevolving.com/2015/03/abap-740-table-expressions-read-modif-itab-line/