In the mesh structure as of ABAP 740, you can move forward or backward using the association. Lets check out more on them.
Introduction
In the last article, you have seen how to define the MESH and the association. If you have not read that article yet, you need to read it before continuing to understand about mesh. Mesh would have an association – a condition – using which you can access the different tables. There could be forward (default) or inverse association.
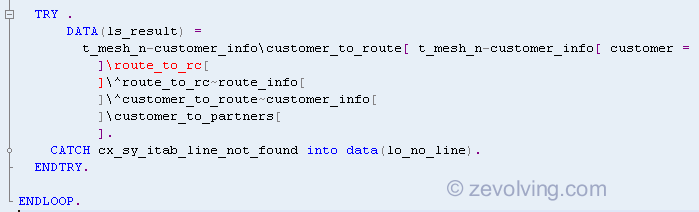
Multiple Associations
You can chain the association together. The default association is the forward association.
DATA(ls_route) = t_mesh-order_info\order_to_customer[ t_mesh-order_info[ order = ls_order-order ] ]\customer_to_route[ ] .
The first association has to have the key to point it into the mesh – something like a starting point. All other subsequent associations can be without the condition.
Note that the mesh node is also accessed with the square bracket sign [ ] as Tabular expression and the old ITAB[] to show the body. The difference here is there has to be space between the brackets. If you don’t have the space, it would give you the error “No Component Exist with the name ..”
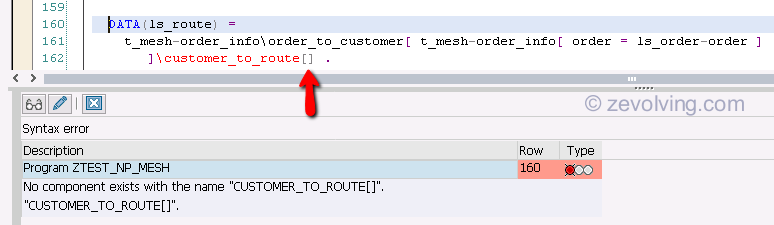
Forward association
The example in the article shows the forward association. To go forward in the mesh, you need to chain together multiple associations in the mesh path. Like:
data(ls_result) = t_mesh_n-customer_info\customer_to_route[ t_mesh_n-customer_info[ customer = ls_cust_n-customer ] ]\route_to_rc[ ]\rc_to_types [ ]\types_to_desc[ ].
Here you have multiple forward associations using the association names – customer_to_route > route_to_rc > rc_to_types > types_to_desc .
Inverse Association
The inverse association is to go back one step on the mesh path. To use the inverse association, you need to use it with ^ in front of the association name. Also, you need to specify the node name with the association with connection ~. This specification is required as the source node can be a target node of different association. Like:
DATA(lt_customers) = t_mesh-order_info\order_to_customer[ t_mesh-order_info[ order = ls_order-order ] ]\customer_to_route[ ]\^customer_to_route~customer_info[ ].
So, in here you are going from order_to_customer > customer_to_route. With ^customer_to_route~customer_info[ ] going back to the customer node, which is the target of the association order_to_customer.
Example 1 – Forward and Inverse association
The association is like customer_to_route > route_to_rc > back to route_info > back to customer_info > customer_to_partner. Visually looks this:
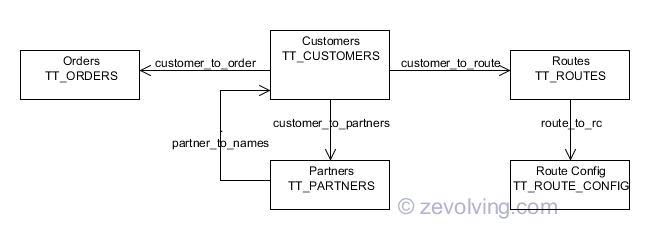
REPORT ztest_np_mesh. TYPES: BEGIN OF ty_customer, customer TYPE char10, name TYPE char30, city TYPE char30, route TYPE char10, END OF ty_customer. TYPES: tt_customers TYPE SORTED TABLE OF ty_customer WITH UNIQUE KEY customer. TYPES: BEGIN OF ty_orders, order TYPE char10, customer TYPE char10, END OF ty_orders. TYPES: tt_orders TYPE SORTED TABLE OF ty_orders WITH UNIQUE KEY order. TYPES: BEGIN OF ty_routes, route TYPE char10, name TYPE char40, END OF ty_routes. TYPES: tt_routes TYPE SORTED TABLE OF ty_routes WITH UNIQUE KEY route. TYPES: BEGIN OF ty_partners, customer TYPE char10, p_type TYPE c LENGTH 2, partner TYPE char10, END OF ty_partners. TYPES: tt_partners TYPE SORTED TABLE OF ty_partners WITH UNIQUE KEY customer p_type. * TYPES: BEGIN OF ty_route_config, route TYPE char10, c_type TYPE char10, c_value TYPE char40, END OF ty_route_config. TYPES: tt_route_config TYPE SORTED TABLE OF ty_route_config WITH UNIQUE KEY route c_type. TYPES: BEGIN OF MESH ty_rep_mesh_n, customer_info TYPE tt_customers ASSOCIATION customer_to_order TO order_info ON customer = customer ASSOCIATION customer_to_route TO route_info ON route = route ASSOCIATION customer_to_partners TO partners ON customer = customer, partners TYPE tt_partners ASSOCIATION partner_to_names TO customer_info ON customer = partner, order_info TYPE tt_orders, route_info TYPE tt_routes ASSOCIATION route_to_rc TO route_config ON route = route, route_config TYPE tt_route_config, END OF MESH ty_rep_mesh_n. DATA(t_customres) = VALUE tt_customers( ( customer = 'C0001' name = 'Test Customer 1' city = 'NY' route = 'R0001' ) ( customer = 'C0002' name = 'Customer 2' city = 'LA' route = 'R0003' ) ( customer = 'C0003' name = 'Good Customer 3' city = 'DFW' route = 'R0001' ) ( customer = 'C0004' name = 'Best Customer 4' city = 'CH' route = 'R0003' ) ). DATA(t_partners) = VALUE tt_partners( ( customer = 'C0001' p_type = 'PY' partner = 'P0001' ) ( customer = 'C0001' p_type = 'SH' partner = 'S0001' ) ( customer = 'C0002' p_type = 'PY' partner = 'P0002' ) ( customer = 'C0002' p_type = 'SH' partner = 'S0002' ) ( customer = 'C0003' p_type = 'PY' partner = 'P0003' ) ( customer = 'C0004' p_type = 'SH' partner = 'S0004' ) ). DATA(t_orders) = VALUE tt_orders( ( order = '90001' customer = 'C0001' ) ( order = '90002' customer = 'C0002' ) ( order = '90003' customer = 'C0001' ) ( order = '90004' customer = 'C0001' ) ( order = '90005' customer = 'C0004' ) ( order = '90006' customer = 'C0004' ) ( order = '90007' customer = 'C0003' ) ). DATA(t_routes) = VALUE tt_routes( ( route = 'R0001' name = 'Route 1' ) ( route = 'R0002' name = 'Route 2' ) ( route = 'R0003' name = 'Route 3' ) ). DATA(t_rc) = VALUE tt_route_config( ( route = 'R0001' c_type = 'RTYPE' c_value = 'DSD' ) ( route = 'R0001' c_type = 'MAX' c_value = '10' ) ( route = 'R0002' c_type = 'RTYPE' c_value = 'WH' ) ( route = 'R0002' c_type = 'MAX' c_value = '100' ) ( route = 'R0003' c_type = 'RTYPE' c_value = 'WH' ) ( route = 'R0004' c_type = 'RTYPE' c_value = 'DSD' ) ). DATA t_mesh_n TYPE ty_rep_mesh_n. t_mesh_n-order_info = t_orders. t_mesh_n-customer_info = t_customres. t_mesh_n-partners = t_partners. t_mesh_n-route_info = t_routes. t_mesh_n-route_config = t_rc. LOOP AT t_mesh_n-customer_info INTO DATA(ls_cust_n). WRITE: / ls_cust_n-customer. WRITE: / ' Orders'. LOOP AT t_mesh_n-customer_info\customer_to_order[ t_mesh_n-customer_info[ customer = ls_cust_n-customer ] ] INTO DATA(ls_order_n). WRITE: / ls_order_n. ENDLOOP. TRY . DATA(ls_result) = t_mesh_n-customer_info\customer_to_route[ t_mesh_n-customer_info[ customer = ls_cust_n-customer ] ]\route_to_rc[ ]\^route_to_rc~route_info[ ]\^customer_to_route~customer_info[ ]\customer_to_partners[ ]. WRITE: / ' partner', ls_result-partner. CATCH cx_sy_itab_line_not_found into data(lo_no_line). write: / 'not found', lo_no_line->get_text( ). ENDTRY. ENDLOOP.
This would generate the output like this:
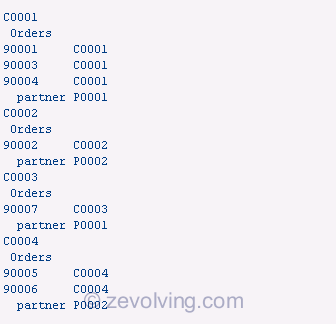
Example 2 – Forward association with condition
Also notice that the first association has the starting point, but rest all of them don’t have any key. You can add the key if you are looking for something very specific. Otherwise, you don’t need to specify the key.
data(ls_route_n) = t_mesh_n-customer_infocustomer_to_route[ t_mesh_n-customer_info[ customer = ls_cust_n-customer ] ]route_to_rc[ c_type = 'MAX' ].
With the condition the output is like this
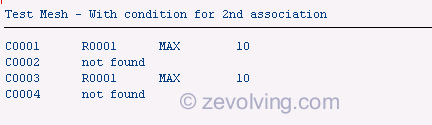
Where as Without the condition the output is like
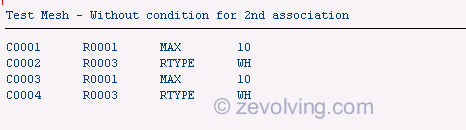
Example 3 – Missing data for condition in the association
What happens when there is some data missing in the association? It will stop there and raise the exception that entry is not found, even the entry is there in the follow on tables. Sweet !!
DATA(t_rc) = VALUE tt_route_config( ( route = 'R0001' c_type = 'RTYPE' c_value = 'DSD' ) ( route = 'R0001' c_type = 'MAX' c_value = '10' ) ( route = 'R0002' c_type = 'RTYPE' c_value = 'WH' ) ( route = 'R0002' c_type = 'MAX' c_value = '100' ) " ( route = 'R0003' c_type = 'RTYPE' c_value = 'WH' ) " ( route = 'R0004' c_type = 'RTYPE' c_value = 'DSD' ) ).
Output of the same loop with same chained association the main example, would produce this output:
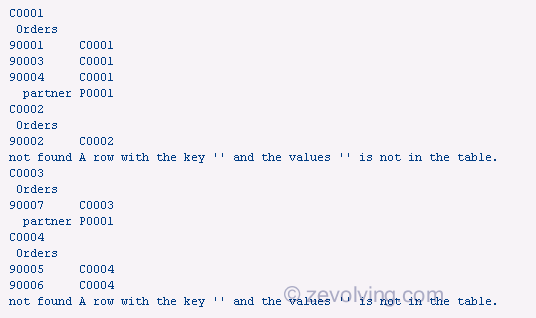
Example 4 – Get all matching values using FOR
This example will get all the orders for customer C0001. Notice the use of FOR here. More on FOR in the next article.
DATA(lt_orders) = VALUE tt_orders( FOR <root> IN t_mesh_n-customer_info WHERE ( customer = 'C0001' ) FOR <node> IN t_mesh_n-customer_infocustomer_to_order[ <root> ] ( <node> ) ).
Table of Content – ABAP 740 Concepts
- ABAP 740 – NEW Operator to instantiate the objects
- ABAP 740 – NEW Operator to create ITAB entries
- ABAP 740 – VALUE Operator to create ITAB entries
- ABAP 740 – Table Expressions to Read & Modify ITAB line
- ABAP 740 – LINE_EXISTS to check record in ITAB
- ABAP 740 – Meshes – A new complex type of Structures
- ABAP 740 – Mesh Path – Forward and Inverse Association
- ABAP 740 – FOR Iteration Expression
- ABAP 740 SWITCH – Conditional Operator
- ABAP 740 – LOOP AT with GROUP BY
- ABAP 740 – Is CONSTANT not a Static Attribute anymore?
- SALV IDA (Integrated Data Access) – Introduction
- SALV IDA – Selection Conditions
- SALV IDA – New Calculated Fields
- SALV IDA – Column Settings
- SALV IDA – Add and Handle Hotspot (Hyperlink)
Too cool!!! And again I’ll have to wait until we upgrade.
Thanks Naimesh, but I’ll really be thankful next year…
Thanks Naimesh.. Very nice post.
I think you can use this concept in internal table join related operations..