Similar to the NEW operator to instantiate the object, you can use the NEW as well with internal table processing to append rows within it.
Preface
In ABAP 740, we have new NEW operator to create the table entries. This NEW works similarly like NEW operator for Object Creation. Lets take a look at what how NEW can be used.
Using NEW operator, the entries within internal table can be created with less coding. Something like this:

When you use the NEW operator to create the Internal table (ITAB) entries, you would get the reference of the data object which holds those values.
Rule of thumb to use NEW would be:
Start a new row with opening bracket (,
Specify the name of the component and relevant value,
When done with that particular row finish it with closing bracket )
Example 1 – Standard table with Component as TABLE_LINE
Here we have a standard table with TABLE_LINE as the component of type I. Without using the NEW operator, you would need to
- Create the data object
- Assign the reference to Field Symbols to work with
- Append the entries to the Field Symbol, which would be added back to the data object
Compare the coding for both classical and using the NEW operator
*---- * Example 1 *---- TYPES t_itab TYPE STANDARD TABLE OF i WITH DEFAULT KEY . * classical DATA dref_o TYPE REF TO data. FIELD-SYMBOLS <fs_o> TYPE t_itab. CREATE DATA dref_o TYPE t_itab. ASSIGN dref_o->* TO <fs_o>. APPEND: 100 TO <fs_o>, 0 TO <fs_o>, 300 TO <fs_o>. * using New DATA dref TYPE REF TO data. dref = NEW t_itab( ( 100 ) ( ) ( 300 ) ).
In debug mode to see the values of both variables:
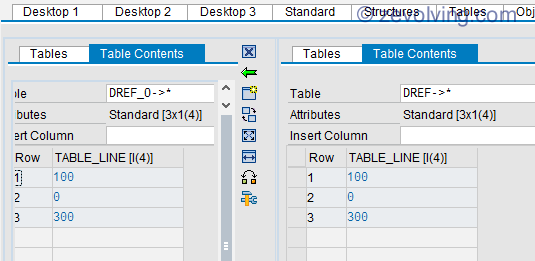
Example 2 – Sorted table with component as TABLE_LINE
When working with the sorted table with classical approach, you need to read the entry and use the SY-TABIX to insert the entry at its own logical location among those entries. The NEW operator also works well with the SORTED table. The entry would be inserted in the sorted table based on its logical sequence, even if you pass them in different order. Easy, huh?
*---- * Example 2 *---- TYPES t_itab_sorted TYPE SORTED TABLE OF i WITH UNIQUE KEY table_line. * classical DATA dref_s_o TYPE REF TO data. FIELD-SYMBOLS <ft_s_o> TYPE t_itab_sorted. CREATE DATA dref_s_o TYPE t_itab_sorted. ASSIGN dref_s_o->* TO <ft_s_o>. READ TABLE <ft_s_o> TRANSPORTING NO FIELDS WITH KEY table_line = 100. IF sy-subrc NE 0. INSERT 100 INTO <ft_s_o> INDEX sy-tabix. ENDIF. READ TABLE <ft_s_o> TRANSPORTING NO FIELDS WITH KEY table_line = 0. IF sy-subrc NE 0. INSERT 0 INTO <ft_s_o> INDEX sy-tabix. ENDIF. READ TABLE <ft_s_o> TRANSPORTING NO FIELDS WITH KEY table_line = 300. IF sy-subrc NE 0. INSERT 300 INTO <ft_s_o> INDEX sy-tabix. ENDIF. * Using new DATA(dref_sorted) = NEW t_itab_sorted( ( 100 ) ( ) ( 300 ) ).
Checking in debug to verify the values in both sorted table
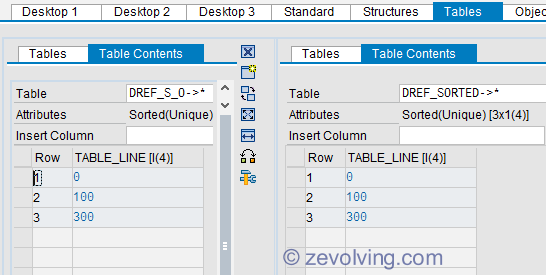
Example 3 – Sorted table with a specific component
I guess you would now got the hang of it on using the NEW. In this example, we would now use the single component in the sorted table.
*---- * Example 3 *---- TYPES: BEGIN OF ty_sorted, num TYPE i, END OF ty_sorted, tt_sorted TYPE SORTED TABLE OF ty_sorted WITH UNIQUE KEY num. * Omitted the classical approach as it would be similar to Example 2 * Using New DATA(dref_sorted_c) = NEW tt_sorted( ( num = 100 ) ( ) ( num = 300 ) ).
In previous two examples, we didn’t specify the component as there was no component in the NEW statement. But, when you have component, you need to specify the component. If you don’t specify, you would get this error. However, that doesn’t mean that you need to specify the component name NUM when you don’t have value, see the second row.
The type of “100” cannot be converted to the type of “TY_SORTED”.
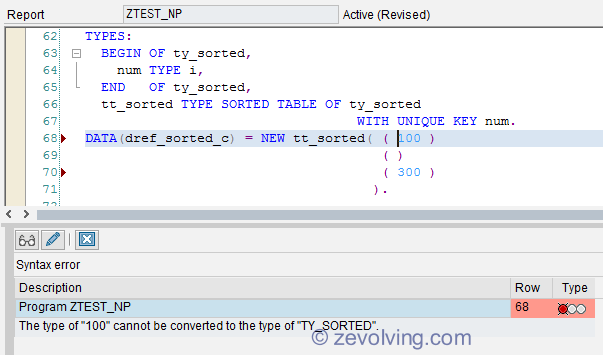
Example 4 – Table with more components
In this example, we would compare working with multiple components. It is same as example 3, Start a new row with opening baracket (, specify the name of the component and relevant value. When done with that particular row finish it with closing bracket ).
* Example 4 TYPES: BEGIN OF ty_data, kunnr TYPE kunnr, name1 TYPE name1, ort01 TYPE ort01, land1 TYPE land1, END OF ty_data. TYPES: tt_data TYPE STANDARD TABLE OF ty_data WITH DEFAULT KEY. * classical DATA: o_data_multi_c TYPE REF TO data. FIELD-SYMBOLS: <ft> TYPE tt_data, <fs> LIKE LINE OF <ft>. CREATE DATA o_data_multi_c TYPE tt_data. ASSIGN o_data_multi_c->* TO <ft>. APPEND INITIAL LINE TO <ft> ASSIGNING <fs>. <fs>-kunnr = '123'. <fs>-name1 = 'ABCD'. <fs>-ort01 = 'LV'. <fs>-land1 = 'NV'. APPEND INITIAL LINE TO <ft> ASSIGNING <fs>. <fs>-kunnr = '456'. <fs>-name1 = 'XYZ'. <fs>-ort01 = 'LA'. <fs>-land1 = 'CA'. * Using New DATA(o_data_multi_comp) = NEW tt_data( ( kunnr = '123' name1 = 'ABCD' ort01 = 'LV' land1 = 'NV' ) ( kunnr = '456' name1 = 'XYZ' ort01 = 'LA' land1 = 'CA' ) ).
In debug mode to compare the values
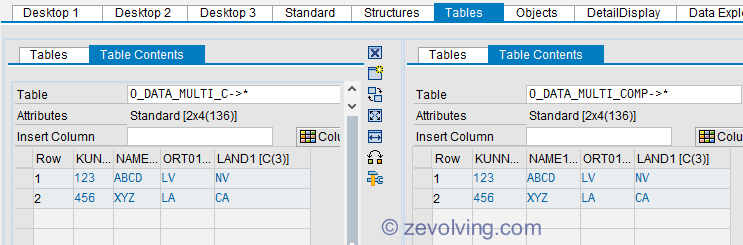
Example 5 – Table with Deep structure
Things get more interesting when you have deep structures. When you work with deep tables, you need to have helper workareas or Field Symbols to insert the entries. But, using NEW operator, you can eliminate the need for it.
To fill up the deep part of the structure T_COLOR, I have used VALUE. Read more about the VALUE operator to create new entries.
* Example 5 TYPES: BEGIN OF ty_alv_data, kunnr TYPE kunnr, name1 TYPE name1, ort01 TYPE ort01, land1 TYPE land1, t_color TYPE lvc_t_scol, END OF ty_alv_data. TYPES: tt_alv_data TYPE STANDARD TABLE OF ty_alv_data WITH DEFAULT KEY. * classical DATA: o_alv_data_c TYPE REF TO data. FIELD-SYMBOLS: <ft_a> TYPE tt_alv_data, <fs_a> LIKE LINE OF <ft_a>, <fs_col> LIKE LINE OF <fs_a>-t_color. CREATE DATA o_alv_data_c TYPE tt_alv_data. ASSIGN o_alv_data_c->* TO <ft_a>. APPEND INITIAL LINE TO <ft_a> ASSIGNING <fs_a>. <fs_a>-kunnr = '123'. <fs_a>-name1 = 'ABCD'. <fs_a>-ort01 = 'LV'. <fs_a>-land1 = 'NV'. APPEND INITIAL LINE TO <fs_a>-t_color ASSIGNING <fs_col>. <fs_col>-fname = 'KUNNR'. <fs_col>-color-col = col_negative. <fs_col>-color-int = 0. <fs_col>-color-inv = 0. APPEND INITIAL LINE TO <fs_a>-t_color ASSIGNING <fs_col>. <fs_col>-fname = 'ORT01'. <fs_col>-color-col = col_total. <fs_col>-color-int = 1. <fs_col>-color-inv = 1. APPEND INITIAL LINE TO <ft_a> ASSIGNING <fs_a>. <fs_a>-kunnr = '456'. <fs_a>-name1 = 'XYZ'. <fs_a>-ort01 = 'LA'. <fs_a>-land1 = 'CA'. DATA(o_alv_data) = NEW tt_alv_data( "First Row ( kunnr = '123' name1 = 'ABCD' ort01 = 'LV' land1 = 'NV' " color table t_color = VALUE #( " Color table - First Row ( fname = 'KUNNR' color-col = col_negative color-int = 0 color-inv = 0 ) " Color Table - 2nd Row ( fname = 'ORT01' color-col = col_total color-int = 1 color-inv = 1 ) ) ) "Second row ( kunnr = '456' name1 = 'XYZ' ort01 = 'LA' land1 = 'CA' ) ).
Again in debug:
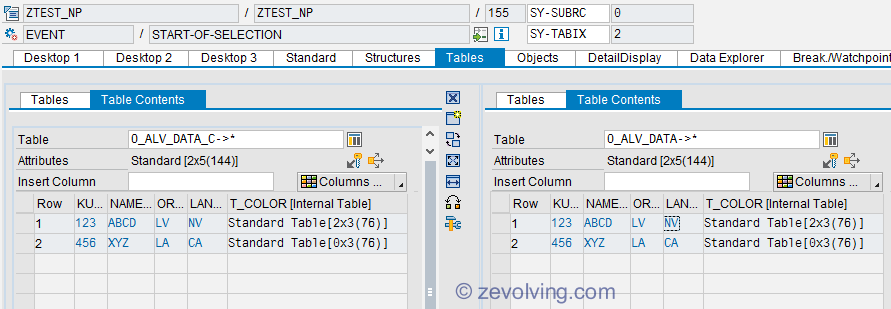
Example 6 – Sorted table with duplicate values
If you try to violate the rules while appending the values in the SORTED table, system will produce the runtime error as expected. Appending the new values follow the same rules as INSERT.
TYPES: BEGIN OF ty_sorted, num TYPE i, END OF ty_sorted, tt_sorted TYPE SORTED TABLE OF ty_sorted WITH UNIQUE KEY num. DATA(itab_sorted_c) = VALUE tt_sorted( ( num = 100 ) ( num = 300 ) ( num = 100 ) ).
Runtime error as expected:
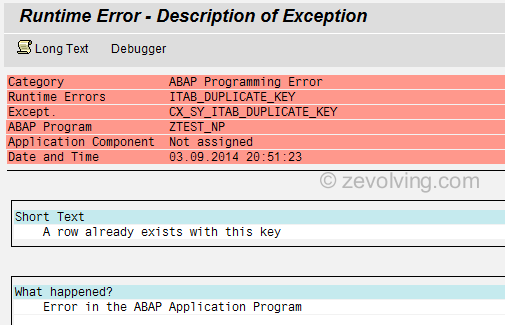
Table without Explicit Key definition
If you omit the key specification, you can’t use the NEW specification to the table – You need to declare table with specify the key type.
A value of the generic type “T_ITAB” cannot be constructed.
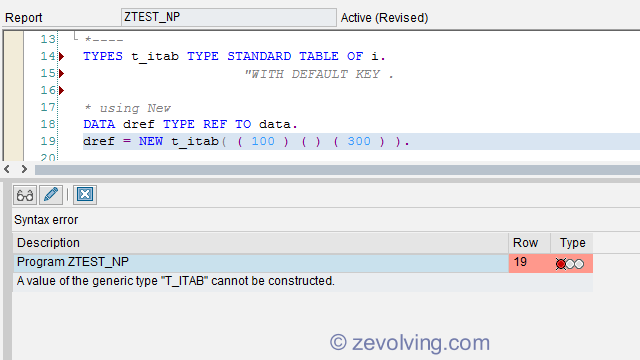
Would you use this or stay with CREATE DATA?
Table of Content – ABAP 740 Concepts
- ABAP 740 – NEW Operator to instantiate the objects
- ABAP 740 – NEW Operator to create ITAB entries
- ABAP 740 – VALUE Operator to create ITAB entries
- ABAP 740 – Table Expressions to Read & Modify ITAB line
- ABAP 740 – LINE_EXISTS to check record in ITAB
- ABAP 740 – Meshes – A new complex type of Structures
- ABAP 740 – Mesh Path – Forward and Inverse Association
- ABAP 740 – FOR Iteration Expression
- ABAP 740 SWITCH – Conditional Operator
- ABAP 740 – LOOP AT with GROUP BY
- ABAP 740 – Is CONSTANT not a Static Attribute anymore?
- SALV IDA (Integrated Data Access) – Introduction
- SALV IDA – Selection Conditions
- SALV IDA – New Calculated Fields
- SALV IDA – Column Settings
- SALV IDA – Add and Handle Hotspot (Hyperlink)
Loving the new syntax, just waiting until we can get on a release of SAP which will support it.
How are errors shown?
Can you give an example what happens,
if you try to put twice the same key in a sorted table?
NEW t_itab( ( 100 ) ( ) ( 300 ) ).
NEW t_itab( ( 100 ) ).
@Markus:
in your example you will not get an error because you are creating a second table.
Only if you try “NEW t_itab( ( 100 ) ( 100 ) ( 300 ) ).” you’ll get an CX_SY_ITAB_DUPLICATE_KEY exception.
I thought that “NEW t_itab( ( 100 ) ( ) ( 300 ) ).” will also throw an exception (not sorted), but it works, the table contains 0, 100, 300 in sorted order.
@Uwe thx! I actually dont have a 7.40, but I would love to … 🙂
Naimesh said: “When you use the NEW operator to create the Internal table or ITAB entries”.
DId I get him wrong, that I can use the NEW Operator for new lines in the Itab, too ?
@Markus:
No, you only can initialize internal tables, not append new lines. See also the examples for the VALUE operator http://scn.sap.com/community/abap/blog/2013/05/27/abap-news-for-release-740–constructor-operator-value
Hello Markus/Uwe,
I wanted to use the OR for Internal table and ITAB, but not with entries. It should read as:
“NEW operator to create the Internal table (ITAB) entries”
Sorry for confusion and Thanks for finding it 🙂 I have corrected it.
I will also add the screenshot for the short dump when there is a duplicate entry for sorted table.
I’m planning to write about the VALUE operator in next article.
Thanks,
Naimesh Patel
@Markus,
I have added example 6 to illustrate the duplicate key runtime error CX_SY_ITAB_DUPLICATE_KEY.
Hi Naimesh,
Good post.
There are many such new options like( which u might already know )
Reading index – ls_wa = itab[ 1 ].
check if line exists – if line_exists( itab [ fieldname = fieldvalue ] ).
assigning field-symbol – assign itab[ fieldname = fieldvalue] to field-symbol( ).
casting operator ?= – obj1 = cast #( obj2 ).
Also, with the introduction of core data services( applicable to all db’s supported by SAP 🙂 and new open sql, its really interesting nowadays. I am lucky that I am working on the latest system 🙂
Regards
Kesav
Hello Keshav,
Glad that you like the post.
Yes, there are many new things in 740. I’m aware of what you have mentioned, but they are still more which I need to get hands on. As 740 is not “primary” system at my client, I’m on exploration journey when time permits.
Do let me know if you come across more fun and useful things.
Thanks.
Hi Naimesh,
In Example 2 classical you don’t need this long syntax.
You can use:
INSERT 300 INTO TABLE .
‘INTO TABLE’ finds the right place in the sorted table. In SY-SUBRC you can check duplicate key error.
Regards,
Pál